Hello! I’m trying to get the Qwiic Mux 1.1 working for the first time with the RedBoard (Arduino Uno) and two sets of Qwiic Alphanumeric Displays. I have verified that the displays work connected directly to the RedBoard, with their jumpers set so I can access four at a time connected in a chain. My end goal is to access two chains via the mux.
I’m currently stuck here:
myMux.begin()
succeeds.
- Prior to setting a port,
myMux.getPort()
returns 255.
- After
myMux.setPort(0)
, myMux.getPort()
returns 255.
- Attempting to
begin()
a device on port 0 after myMux.setPort(0)
fails (returns false).
Hookup photos and sketch code below. What’d I miss?
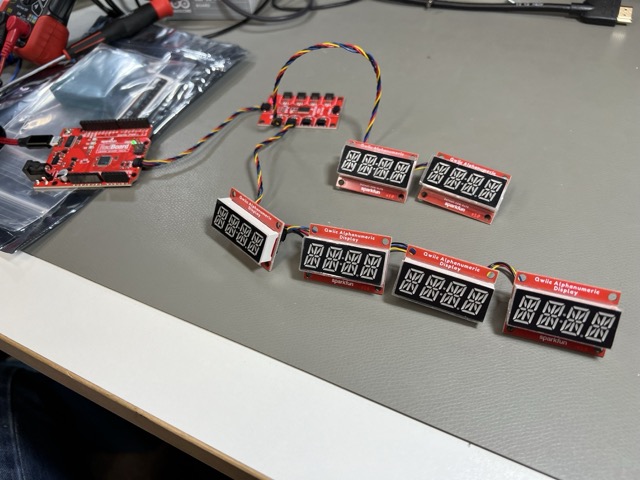
#include <Wire.h>
#include <SparkFun_I2C_Mux_Arduino_Library.h>
#include <SparkFun_Alphanumeric_Display.h>
QWIICMUX myMux;
HT16K33 addrDisplay; // four digits
HT16K33 memDisplay; // eight digits
void setup()
{
// Serial output back to the PC for debugging.
Serial.begin(115200);
Serial.println("Hello.");
// Join the I2C bus.
Wire.begin();
if (myMux.begin() == false)
{
Serial.println("Mux not detected.");
while(1);
}
for (int p=0; p<7; p++) {
myMux.disablePort(p);
}
byte currentPortNumber = myMux.getPort();
Serial.print("CurrentPort: ");
Serial.println(currentPortNumber); // This is printing 255.
myMux.setPort(0);
currentPortNumber = myMux.getPort();
Serial.print("CurrentPort: ");
Serial.println(currentPortNumber); // This is printing 255.
if (addrDisplay.begin(0x70, 0x71) == false) // This is returning false.
{
Serial.println("Address display not detected.");
while (1);
}
addrDisplay.print("ABCDEFGH");
myMux.setPort(1);
if (memDisplay.begin(0x70, 0x71, 0x72, 0x73) == false)
{
Serial.println("Memory display not detected.");
while (1);
}
memDisplay.print("0123456789ABCDEF");
}
void loop()
{
}
Simplifying:
One display with default jumpers (address 0x70) connected directly to the RedBoard works fine:
if (addrDisplay.begin(0x70) == false)
{
Serial.println("Address display not detected.");
while (1);
}
Serial.println("Address display detected.");
addrDisplay.print("AXYZ"); // Displays
The same display connected to port 0 on the mux, and the mux connected to the RedBoard, with nothing else connected, displays nothing, but also the begin()
s both succeed. But if I disconnect the display from the mux, its begin()
still succeeds. Maybe I’m misunderstanding how the mux uses addresses?
if (myMux.begin() == false)
{
Serial.println("Mux not detected.");
while(1);
}
Serial.println("Mux detected.");
myMux.setPort(0);
if (addrDisplay.begin(0x70) == false)
{
Serial.println("Address display not detected.");
while (1);
}
Serial.println("Address display detected.");
addrDisplay.print("AXYZ");
Disable the i2c pull-up resistors on all but on device (I usually recommend leaving the mux’s intact and disabling the screens’); scroll up 2-3 paragraphs here
Also run through the mux guide examples (but substitute the qwiic alphanumeric code instead of the accelerometer code)
Note that you can also set up to 4 individual addresses on the qwiic alphas by modifying their address jumper without using a mux at all (still need to disable all but one set of i2c pull up resistors though)
Thanks for the reply! I located the three pads for the pull-up resistors on the display, and used the x-acto knife to cut the traces between the three pads, and tested to confirm there is no electrical continuity between them. (I also compared with an uncut display to confirm.) This is still not working when connected to mux port 0, using the simplified code in my latter post. Does it sound like I made the correct modification?
I’m hoping to use six of the displays (a set of two and a set of four), so I originally thought I’d be using two chains (0x70-0x71 and 0x70-0x73). I could connect all six directly to the mux if necessary, once I get the first display running through the mux.
The mux Example1_BasicControl detects an I2C device at address 0x70 with only the mux connected. When I connect a display configured for address 0x70 to port 0 of the mux, it still only reports one device at address 0x70.
I feel like I’m misunderstanding how the mux operates. The mux itself is listening on I2C address 0x70, yes? If I tell it to select port 0, what address do I use for messages intended for the device on port 0? How does the mux know what address the device itself is listening for, to route messages?
Does the mux need to be jumpered to an address not in use by the devices on the active port? Can I communicate with devices jumpered for 0x70-0x73 all on port 0 via the mux, or do all four devices need to be on separate mux ports, configured to some address?
(Apologies for the multiple replies, I’m figuring it out.
)
Ah, so if I connect the string of displays 0x71-0x73 to port 1 then run the first example, it reports there are devices on 0x70-0x73. So I think I’m now understanding that the address of the mux itself cannot conflict with any of the addresses on the selected port. One possible solution is to jumper the mux to 0x74, which is not used by any of the displays. Another solution is to jumper all the displays to use 0x71 and put them on separate ports, and use 0x70 for the mux.
I’ll give this a try. lmk if I’m still missing something. 
That was it!
Summarizing for future searchers: Both the Qwiic mux and the Qwiic alphanumeric display use address 0x70 by default. To use an alpha display with the mux, one of them must be reconfigured to use a different address by adding solder to an address jumper. In my case, I reconfigured the mux to use 0x74, so that my displays could use addresses 0x70 to 0x73. Messages reach the mux and everything on the mux’s active port. By design, devices on separate ports can reuse addresses, but they cannot use the address used by the mux itself.
I also took Russell’s advice and used a craft knife to cut the pull-up resistor jumper pad traces on all of the displays. The mux provides the pull-up resistors needed for the I2C bus to the RedBoard.
1 Like
Excellent! I did not notice that when I was skimming through the docs, good catch & follow-up 